Managing Applications and Infrastructure with Terraform-Deploying Infrastructure with Terraform-(2)Terraform for AWS-(15)Terraform Formatting and Remote State
2018年10月04日
Rewrite Terraform configuration files to a canonical format and style.
ec2-user:~/environment/AWS $ terraform fmt --diff
Now we try to store the Terraform state files onto S3 instead of a machine locally.
Create an S3 bucket, la-terraform-course-state-sc.
ec2-user:~/environment/AWS $ aws s3 ls | grep la-terraform-course-state-sc
Create a prefix called "terraform" in S3 bucket (i.e. "la-terraform-course-state-sc").
Edit the AWS/main.tf file.
ec2-user:~/environment/AWS $ terraform init
ec2-user:~/environment/AWS $ terraform plan
There is currently on objects under the terraform prefix.
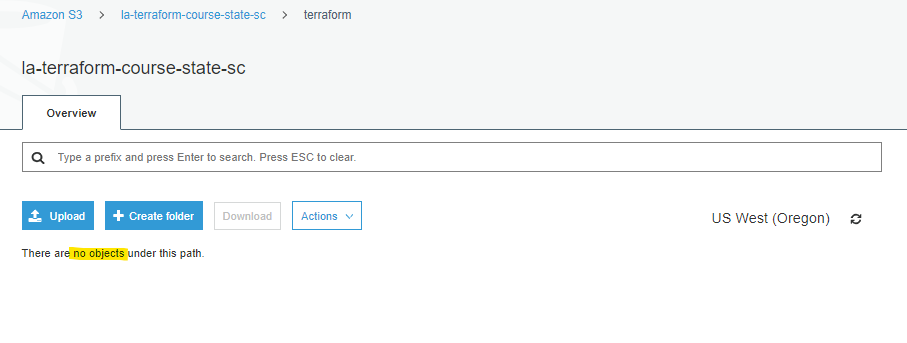
ec2-user:~/environment/AWS $ terraform apply
Object terraform.tfstate appeared under prefix terraform of S3 bucket la-terraform-course-state-sc.

ec2-user:~/environment/AWS $ ls
ec2-user:~/environment/AWS $ terraform show
ec2-user:~/environment/AWS $ mv terraform.tfstate terraform.tfstate.bak
ec2-user:~/environment/AWS $ terraform show
Rename object terraform.tfstate to terraform.tfstate.bak
ec2-user:~/environment/AWS $ terraform show
No state.
*
ec2-user:~/environment/AWS $ mv terraform.tfstate.bak terraform.tfstate
ec2-user:~/environment/AWS $ terraform show
No state.
*
Rename object terraform.tfstate.bak back to terraform.tfstate
ec2-user:~/environment/AWS $ terraform show
ec2-user:~/environment/AWS $ terraform destroy
References
Command: fmt
Managing Applications and Infrastructure with Terraform-Deploying Infrastructure with Terraform
2. Terraform for AWS
15. Terraform Formatting and Remote State
ec2-user:~/environment/AWS $ terraform destroy
ec2-user:~/environment/AWS $ ls
compute networking storage terraform.tfstate.backup variables.tf main.tf outputs.tf terraform.tfstate terraform.tfvars
Rewrite Terraform configuration files to a canonical format and style.
ec2-user:~/environment/AWS $ terraform fmt --diff
main.tf --- old/main.tf +++ new/main.tf @@ -1,29 +1,29 @@ provider "aws" { - region = var.aws_region + region = var.aws_region } # Deploy Storage Resource module "storage" { - source = "./storage" - project_name = var.project_name + source = "./storage" + project_name = var.project_name } # Deploy Networking Resources module "networking" { - source = "./networking" - vpc_cidr = var.vpc_cidr - public_cidrs = var.public_cidrs - accessip = var.accessip + source = "./networking" + vpc_cidr = var.vpc_cidr + public_cidrs = var.public_cidrs + accessip = var.accessip } # Deploy Compute Resources module "compute" { - source = "./compute" - instance_count = var.instance_count - key_name = var.key_name - public_key_path = var.public_key_path - instance_type = var.server_instance_type - subnets = module.networking.public_subnets - security_group = module.networking.public_sg - subnet_ips = module.networking.subnet_ips + source = "./compute" + instance_count = var.instance_count + key_name = var.key_name + public_key_path = var.public_key_path + instance_type = var.server_instance_type + subnets = module.networking.public_subnets + security_group = module.networking.public_sg + subnet_ips = module.networking.subnet_ips } \ No newline at end of file outputs.tf --- old/outputs.tf +++ new/outputs.tf @@ -4,29 +4,29 @@ #---storage outputs output "Bucket_Name" { - value = module.storage.bucketname + value = module.storage.bucketname } #---Networking Outputs output "Public_Subnets" { - value = join(", ", module.networking.public_subnets) + value = join(", ", module.networking.public_subnets) } output "Subnet_IPs" { - value = join(", ", module.networking.subnet_ips) + value = join(", ", module.networking.subnet_ips) } output "Public_Security_Group" { - value = module.networking.public_sg + value = module.networking.public_sg } #---Compute Outputs output "Public_Instance_IDs" { - value = module.compute.server_id + value = module.compute.server_id } output "Public_Instance_IPs" { - value = module.compute.server_ip + value = module.compute.server_ip } \ No newline at end of file terraform.tfvars --- old/terraform.tfvars +++ new/terraform.tfvars @@ -1,12 +1,12 @@ -aws_region = "us-west-2" +aws_region = "us-west-2" project_name = "la-terrafrom" -vpc_cidr = "10.123.0.0/16" +vpc_cidr = "10.123.0.0/16" public_cidrs = [ - "10.123.1.0/24", - "10.123.2.0/24" - ] -accessip = "0.0.0.0/0" -key_name = "tf_key" -public_key_path = "/home/ec2-user/.ssh/id_rsa.pub" + "10.123.1.0/24", + "10.123.2.0/24" +] +accessip = "0.0.0.0/0" +key_name = "tf_key" +public_key_path = "/home/ec2-user/.ssh/id_rsa.pub" server_instance_type = "t2.micro" -instance_count = 2 \ No newline at end of file +instance_count = 2 \ No newline at end of file variables.tf --- old/variables.tf +++ new/variables.tf @@ -8,7 +8,7 @@ variable "vpc_cidr" {} variable "public_cidrs" { - type = list + type = list } variable "accessip" {} @@ -21,5 +21,5 @@ variable "server_instance_type" {} variable "instance_count" { - default = 1 + default = 1 } \ No newline at end of file
Now we try to store the Terraform state files onto S3 instead of a machine locally.
Create an S3 bucket, la-terraform-course-state-sc.
ec2-user:~/environment/AWS $ aws s3 ls | grep la-terraform-course-state-sc
2020-06-27 15:03:31 la-terraform-course-state-sc
Create a prefix called "terraform" in S3 bucket (i.e. "la-terraform-course-state-sc").
Edit the AWS/main.tf file.
provider "aws" { region = var.aws_region } terraform { backend "s3" { bucket = "la-terraform-course-state-sc" key = "terraform/terraform.tfstate" region = "us-west-2" } } # Deploy Storage Resource module "storage" { source = "./storage" project_name = var.project_name } # Deploy Networking Resources module "networking" { source = "./networking" vpc_cidr = var.vpc_cidr public_cidrs = var.public_cidrs accessip = var.accessip } # Deploy Compute Resources module "compute" { source = "./compute" instance_count = var.instance_count key_name = var.key_name public_key_path = var.public_key_path instance_type = var.server_instance_type subnets = module.networking.public_subnets security_group = module.networking.public_sg subnet_ips = module.networking.subnet_ips }
ec2-user:~/environment/AWS $ terraform init
Initializing modules... Initializing the backend... Successfully configured the backend "s3"! Terraform will automatically use this backend unless the backend configuration changes. Initializing provider plugins... - Using previously-installed hashicorp/aws v3.9.0 - Using previously-installed hashicorp/template v2.2.0 - Using previously-installed hashicorp/random v3.0.0 The following providers do not have any version constraints in configuration, so the latest version was installed. To prevent automatic upgrades to new major versions that may contain breaking changes, we recommend adding version constraints in a required_providers block in your configuration, with the constraint strings suggested below. * hashicorp/aws: version = "~> 3.9.0" * hashicorp/random: version = "~> 3.0.0" * hashicorp/template: version = "~> 2.2.0" Terraform has been successfully initialized! You may now begin working with Terraform. Try running "terraform plan" to see any changes that are required for your infrastructure. All Terraform commands should now work. If you ever set or change modules or backend configuration for Terraform, rerun this command to reinitialize your working directory. If you forget, other commands will detect it and remind you to do so if necessary.
ec2-user:~/environment/AWS $ terraform plan
Refreshing Terraform state in-memory prior to plan... The refreshed state will be used to calculate this plan, but will not be persisted to local or remote state storage. module.compute.data.aws_ami.server_ami: Refreshing state... module.networking.data.aws_availability_zones.available: Refreshing state... ------------------------------------------------------------------------ An execution plan has been generated and is shown below. Resource actions are indicated with the following symbols: + create <= read (data resources) Terraform will perform the following actions: # module.compute.data.template_file.user-init[0] will be read during apply # (config refers to values not yet known) <= data "template_file" "user-init" { + id = "57df141e108f0b6cf1703691b1a5a736ca3a01b0eb84e07196d778d405c36d19" + rendered = <<~EOT #!/bin/bash yum install httpd -y echo "Subnet for Firewall: 10.123.1.0/24" >> /var/www/html/index.html service httpd start chkconfig httpd on EOT + template = <<~EOT #!/bin/bash yum install httpd -y echo "Subnet for Firewall: ${firewall_subnets}" >> /var/www/html/index.html service httpd start chkconfig httpd on EOT + vars = { + "firewall_subnets" = "10.123.1.0/24" } } # module.compute.data.template_file.user-init[1] will be read during apply # (config refers to values not yet known) <= data "template_file" "user-init" { + id = "757bacc9f6377916bf38eff5b6acdb9ae2756c56859b9c203d8de26eff4865d4" + rendered = <<~EOT #!/bin/bash yum install httpd -y echo "Subnet for Firewall: 10.123.2.0/24" >> /var/www/html/index.html service httpd start chkconfig httpd on EOT + template = <<~EOT #!/bin/bash yum install httpd -y echo "Subnet for Firewall: ${firewall_subnets}" >> /var/www/html/index.html service httpd start chkconfig httpd on EOT + vars = { + "firewall_subnets" = "10.123.2.0/24" } } # module.compute.aws_instance.tf_server[0] will be created + resource "aws_instance" "tf_server" { + ami = "ami-01fee56b22f308154" + arn = (known after apply) + associate_public_ip_address = (known after apply) + availability_zone = (known after apply) + cpu_core_count = (known after apply) + cpu_threads_per_core = (known after apply) + get_password_data = false + host_id = (known after apply) + id = (known after apply) + instance_state = (known after apply) + instance_type = "t2.micro" + ipv6_address_count = (known after apply) + ipv6_addresses = (known after apply) + key_name = (known after apply) + outpost_arn = (known after apply) + password_data = (known after apply) + placement_group = (known after apply) + primary_network_interface_id = (known after apply) + private_dns = (known after apply) + private_ip = (known after apply) + public_dns = (known after apply) + public_ip = (known after apply) + secondary_private_ips = (known after apply) + security_groups = (known after apply) + source_dest_check = true + subnet_id = (known after apply) + tags = { + "Name" = "tf_server-1" } + tenancy = (known after apply) + user_data = "544105fc76f56380fa17cd0686e033cee5001d87" + volume_tags = (known after apply) + vpc_security_group_ids = (known after apply) + ebs_block_device { + delete_on_termination = (known after apply) + device_name = (known after apply) + encrypted = (known after apply) + iops = (known after apply) + kms_key_id = (known after apply) + snapshot_id = (known after apply) + volume_id = (known after apply) + volume_size = (known after apply) + volume_type = (known after apply) } + ephemeral_block_device { + device_name = (known after apply) + no_device = (known after apply) + virtual_name = (known after apply) } + metadata_options { + http_endpoint = (known after apply) + http_put_response_hop_limit = (known after apply) + http_tokens = (known after apply) } + network_interface { + delete_on_termination = (known after apply) + device_index = (known after apply) + network_interface_id = (known after apply) } + root_block_device { + delete_on_termination = (known after apply) + device_name = (known after apply) + encrypted = (known after apply) + iops = (known after apply) + kms_key_id = (known after apply) + volume_id = (known after apply) + volume_size = (known after apply) + volume_type = (known after apply) } } # module.compute.aws_instance.tf_server[1] will be created + resource "aws_instance" "tf_server" { + ami = "ami-01fee56b22f308154" + arn = (known after apply) + associate_public_ip_address = (known after apply) + availability_zone = (known after apply) + cpu_core_count = (known after apply) + cpu_threads_per_core = (known after apply) + get_password_data = false + host_id = (known after apply) + id = (known after apply) + instance_state = (known after apply) + instance_type = "t2.micro" + ipv6_address_count = (known after apply) + ipv6_addresses = (known after apply) + key_name = (known after apply) + outpost_arn = (known after apply) + password_data = (known after apply) + placement_group = (known after apply) + primary_network_interface_id = (known after apply) + private_dns = (known after apply) + private_ip = (known after apply) + public_dns = (known after apply) + public_ip = (known after apply) + secondary_private_ips = (known after apply) + security_groups = (known after apply) + source_dest_check = true + subnet_id = (known after apply) + tags = { + "Name" = "tf_server-2" } + tenancy = (known after apply) + user_data = "ea5b38a77b74322af7f46802b7e74b3277c7eb0d" + volume_tags = (known after apply) + vpc_security_group_ids = (known after apply) + ebs_block_device { + delete_on_termination = (known after apply) + device_name = (known after apply) + encrypted = (known after apply) + iops = (known after apply) + kms_key_id = (known after apply) + snapshot_id = (known after apply) + volume_id = (known after apply) + volume_size = (known after apply) + volume_type = (known after apply) } + ephemeral_block_device { + device_name = (known after apply) + no_device = (known after apply) + virtual_name = (known after apply) } + metadata_options { + http_endpoint = (known after apply) + http_put_response_hop_limit = (known after apply) + http_tokens = (known after apply) } + network_interface { + delete_on_termination = (known after apply) + device_index = (known after apply) + network_interface_id = (known after apply) } + root_block_device { + delete_on_termination = (known after apply) + device_name = (known after apply) + encrypted = (known after apply) + iops = (known after apply) + kms_key_id = (known after apply) + volume_id = (known after apply) + volume_size = (known after apply) + volume_type = (known after apply) } } # module.compute.aws_key_pair.tf_auth will be created + resource "aws_key_pair" "tf_auth" { + arn = (known after apply) + fingerprint = (known after apply) + id = (known after apply) + key_name = "tf_key" + key_pair_id = (known after apply) + public_key = "ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQCzIeDhLeOVMfyYVV4ePCm/X4uRRmqkqq84TU2VsBDHWtMuFwBbez2ZmAm+WQ5zOyaZC/soSK17R8TsociZ+9wJBWT62aS3H8IHE2UxoakjlucF1QLM81oZaO5R4DCeKVJb0l/XfZ/fQkhYFLNM5622MbHP8MTTwfrbwE1+hjRFJYb0K364NCD0BLdgn+V7kfyEcSRRds8gh8zdiejJxFHRTaq9LRx+AQwFDkQYEYzk6ZGxIasKonCD18OtwAePdFgA1Mlho6Ajh9VyrgYWrEHKmfvDa/Rz7T/cCy5tkzdu5B04HWI7yBEthZeKm9QA8keOj1xU+yMWSNwUhiXGTg85 ec2-user@ip-10-0-0-44.us-west-2.compute.internal" } # module.networking.aws_default_route_table.tf_private_rt will be created + resource "aws_default_route_table" "tf_private_rt" { + default_route_table_id = (known after apply) + id = (known after apply) + owner_id = (known after apply) + route = (known after apply) + tags = { + "Name" = "tf_private" } + vpc_id = (known after apply) } # module.networking.aws_internet_gateway.tf_internet_gateway will be created + resource "aws_internet_gateway" "tf_internet_gateway" { + arn = (known after apply) + id = (known after apply) + owner_id = (known after apply) + tags = { + "Name" = "tf_igw" } + vpc_id = (known after apply) } # module.networking.aws_route_table.tf_public_rt will be created + resource "aws_route_table" "tf_public_rt" { + id = (known after apply) + owner_id = (known after apply) + propagating_vgws = (known after apply) + route = [ + { + cidr_block = "0.0.0.0/0" + egress_only_gateway_id = "" + gateway_id = (known after apply) + instance_id = "" + ipv6_cidr_block = "" + local_gateway_id = "" + nat_gateway_id = "" + network_interface_id = "" + transit_gateway_id = "" + vpc_peering_connection_id = "" }, ] + tags = { + "Name" = "tf_public" } + vpc_id = (known after apply) } # module.networking.aws_route_table_association.tf_public_assoc[0] will be created + resource "aws_route_table_association" "tf_public_assoc" { + id = (known after apply) + route_table_id = (known after apply) + subnet_id = (known after apply) } # module.networking.aws_route_table_association.tf_public_assoc[1] will be created + resource "aws_route_table_association" "tf_public_assoc" { + id = (known after apply) + route_table_id = (known after apply) + subnet_id = (known after apply) } # module.networking.aws_security_group.tf_public_sg will be created + resource "aws_security_group" "tf_public_sg" { + arn = (known after apply) + description = "Used for access to the public instances" + egress = [ + { + cidr_blocks = [ + "0.0.0.0/0", ] + description = "" + from_port = 0 + ipv6_cidr_blocks = [] + prefix_list_ids = [] + protocol = "-1" + security_groups = [] + self = false + to_port = 0 }, ] + id = (known after apply) + ingress = [ + { + cidr_blocks = [ + "0.0.0.0/0", ] + description = "" + from_port = 22 + ipv6_cidr_blocks = [] + prefix_list_ids = [] + protocol = "tcp" + security_groups = [] + self = false + to_port = 22 }, + { + cidr_blocks = [ + "0.0.0.0/0", ] + description = "" + from_port = 80 + ipv6_cidr_blocks = [] + prefix_list_ids = [] + protocol = "tcp" + security_groups = [] + self = false + to_port = 80 }, ] + name = "tf_public_sg" + owner_id = (known after apply) + revoke_rules_on_delete = false + vpc_id = (known after apply) } # module.networking.aws_subnet.tf_public_subnet[0] will be created + resource "aws_subnet" "tf_public_subnet" { + arn = (known after apply) + assign_ipv6_address_on_creation = false + availability_zone = "us-west-2a" + availability_zone_id = (known after apply) + cidr_block = "10.123.1.0/24" + id = (known after apply) + ipv6_cidr_block_association_id = (known after apply) + map_public_ip_on_launch = true + owner_id = (known after apply) + tags = { + "Name" = "tf_public_1" } + vpc_id = (known after apply) } # module.networking.aws_subnet.tf_public_subnet[1] will be created + resource "aws_subnet" "tf_public_subnet" { + arn = (known after apply) + assign_ipv6_address_on_creation = false + availability_zone = "us-west-2b" + availability_zone_id = (known after apply) + cidr_block = "10.123.2.0/24" + id = (known after apply) + ipv6_cidr_block_association_id = (known after apply) + map_public_ip_on_launch = true + owner_id = (known after apply) + tags = { + "Name" = "tf_public_2" } + vpc_id = (known after apply) } # module.networking.aws_vpc.tf_vpc will be created + resource "aws_vpc" "tf_vpc" { + arn = (known after apply) + assign_generated_ipv6_cidr_block = false + cidr_block = "10.123.0.0/16" + default_network_acl_id = (known after apply) + default_route_table_id = (known after apply) + default_security_group_id = (known after apply) + dhcp_options_id = (known after apply) + enable_classiclink = (known after apply) + enable_classiclink_dns_support = (known after apply) + enable_dns_hostnames = true + enable_dns_support = true + id = (known after apply) + instance_tenancy = "default" + ipv6_association_id = (known after apply) + ipv6_cidr_block = (known after apply) + main_route_table_id = (known after apply) + owner_id = (known after apply) + tags = { + "Name" = "tf_vpc" } } # module.storage.aws_s3_bucket.tf_code will be created + resource "aws_s3_bucket" "tf_code" { + acceleration_status = (known after apply) + acl = "private" + arn = (known after apply) + bucket = (known after apply) + bucket_domain_name = (known after apply) + bucket_regional_domain_name = (known after apply) + force_destroy = true + hosted_zone_id = (known after apply) + id = (known after apply) + region = (known after apply) + request_payer = (known after apply) + tags = { + "Name" = "tf_bucket" } + website_domain = (known after apply) + website_endpoint = (known after apply) + versioning { + enabled = (known after apply) + mfa_delete = (known after apply) } } # module.storage.random_id.tf_bucket_id will be created + resource "random_id" "tf_bucket_id" { + b64_std = (known after apply) + b64_url = (known after apply) + byte_length = 2 + dec = (known after apply) + hex = (known after apply) + id = (known after apply) } Plan: 14 to add, 0 to change, 0 to destroy. Changes to Outputs: + Bucket_Name = (known after apply) + Public_Instance_IDs = (known after apply) + Public_Instance_IPs = (known after apply) + Public_Security_Group = (known after apply) + Public_Subnets = (known after apply) + Subnet_IPs = "10.123.1.0/24, 10.123.2.0/24" ------------------------------------------------------------------------ Note: You didn't specify an "-out" parameter to save this plan, so Terraform can't guarantee that exactly these actions will be performed if "terraform apply" is subsequently run.
There is currently on objects under the terraform prefix.
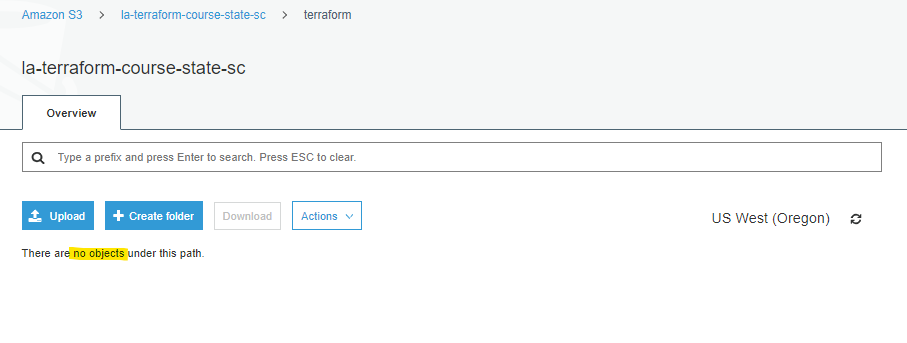
ec2-user:~/environment/AWS $ terraform apply
module.networking.data.aws_availability_zones.available: Refreshing state... module.compute.data.aws_ami.server_ami: Refreshing state... An execution plan has been generated and is shown below. Resource actions are indicated with the following symbols: + create <= read (data resources) Terraform will perform the following actions: # module.compute.data.template_file.user-init[0] will be read during apply # (config refers to values not yet known) <= data "template_file" "user-init" { + id = "57df141e108f0b6cf1703691b1a5a736ca3a01b0eb84e07196d778d405c36d19" + rendered = <<~EOT #!/bin/bash yum install httpd -y echo "Subnet for Firewall: 10.123.1.0/24" >> /var/www/html/index.html service httpd start chkconfig httpd on EOT + template = <<~EOT #!/bin/bash yum install httpd -y echo "Subnet for Firewall: ${firewall_subnets}" >> /var/www/html/index.html service httpd start chkconfig httpd on EOT + vars = { + "firewall_subnets" = "10.123.1.0/24" } } # module.compute.data.template_file.user-init[1] will be read during apply # (config refers to values not yet known) <= data "template_file" "user-init" { + id = "757bacc9f6377916bf38eff5b6acdb9ae2756c56859b9c203d8de26eff4865d4" + rendered = <<~EOT #!/bin/bash yum install httpd -y echo "Subnet for Firewall: 10.123.2.0/24" >> /var/www/html/index.html service httpd start chkconfig httpd on EOT + template = <<~EOT #!/bin/bash yum install httpd -y echo "Subnet for Firewall: ${firewall_subnets}" >> /var/www/html/index.html service httpd start chkconfig httpd on EOT + vars = { + "firewall_subnets" = "10.123.2.0/24" } } # module.compute.aws_instance.tf_server[0] will be created + resource "aws_instance" "tf_server" { + ami = "ami-01fee56b22f308154" + arn = (known after apply) + associate_public_ip_address = (known after apply) + availability_zone = (known after apply) + cpu_core_count = (known after apply) + cpu_threads_per_core = (known after apply) + get_password_data = false + host_id = (known after apply) + id = (known after apply) + instance_state = (known after apply) + instance_type = "t2.micro" + ipv6_address_count = (known after apply) + ipv6_addresses = (known after apply) + key_name = (known after apply) + outpost_arn = (known after apply) + password_data = (known after apply) + placement_group = (known after apply) + primary_network_interface_id = (known after apply) + private_dns = (known after apply) + private_ip = (known after apply) + public_dns = (known after apply) + public_ip = (known after apply) + secondary_private_ips = (known after apply) + security_groups = (known after apply) + source_dest_check = true + subnet_id = (known after apply) + tags = { + "Name" = "tf_server-1" } + tenancy = (known after apply) + user_data = "544105fc76f56380fa17cd0686e033cee5001d87" + volume_tags = (known after apply) + vpc_security_group_ids = (known after apply) + ebs_block_device { + delete_on_termination = (known after apply) + device_name = (known after apply) + encrypted = (known after apply) + iops = (known after apply) + kms_key_id = (known after apply) + snapshot_id = (known after apply) + volume_id = (known after apply) + volume_size = (known after apply) + volume_type = (known after apply) } + ephemeral_block_device { + device_name = (known after apply) + no_device = (known after apply) + virtual_name = (known after apply) } + metadata_options { + http_endpoint = (known after apply) + http_put_response_hop_limit = (known after apply) + http_tokens = (known after apply) } + network_interface { + delete_on_termination = (known after apply) + device_index = (known after apply) + network_interface_id = (known after apply) } + root_block_device { + delete_on_termination = (known after apply) + device_name = (known after apply) + encrypted = (known after apply) + iops = (known after apply) + kms_key_id = (known after apply) + volume_id = (known after apply) + volume_size = (known after apply) + volume_type = (known after apply) } } # module.compute.aws_instance.tf_server[1] will be created + resource "aws_instance" "tf_server" { + ami = "ami-01fee56b22f308154" + arn = (known after apply) + associate_public_ip_address = (known after apply) + availability_zone = (known after apply) + cpu_core_count = (known after apply) + cpu_threads_per_core = (known after apply) + get_password_data = false + host_id = (known after apply) + id = (known after apply) + instance_state = (known after apply) + instance_type = "t2.micro" + ipv6_address_count = (known after apply) + ipv6_addresses = (known after apply) + key_name = (known after apply) + outpost_arn = (known after apply) + password_data = (known after apply) + placement_group = (known after apply) + primary_network_interface_id = (known after apply) + private_dns = (known after apply) + private_ip = (known after apply) + public_dns = (known after apply) + public_ip = (known after apply) + secondary_private_ips = (known after apply) + security_groups = (known after apply) + source_dest_check = true + subnet_id = (known after apply) + tags = { + "Name" = "tf_server-2" } + tenancy = (known after apply) + user_data = "ea5b38a77b74322af7f46802b7e74b3277c7eb0d" + volume_tags = (known after apply) + vpc_security_group_ids = (known after apply) + ebs_block_device { + delete_on_termination = (known after apply) + device_name = (known after apply) + encrypted = (known after apply) + iops = (known after apply) + kms_key_id = (known after apply) + snapshot_id = (known after apply) + volume_id = (known after apply) + volume_size = (known after apply) + volume_type = (known after apply) } + ephemeral_block_device { + device_name = (known after apply) + no_device = (known after apply) + virtual_name = (known after apply) } + metadata_options { + http_endpoint = (known after apply) + http_put_response_hop_limit = (known after apply) + http_tokens = (known after apply) } + network_interface { + delete_on_termination = (known after apply) + device_index = (known after apply) + network_interface_id = (known after apply) } + root_block_device { + delete_on_termination = (known after apply) + device_name = (known after apply) + encrypted = (known after apply) + iops = (known after apply) + kms_key_id = (known after apply) + volume_id = (known after apply) + volume_size = (known after apply) + volume_type = (known after apply) } } # module.compute.aws_key_pair.tf_auth will be created + resource "aws_key_pair" "tf_auth" { + arn = (known after apply) + fingerprint = (known after apply) + id = (known after apply) + key_name = "tf_key" + key_pair_id = (known after apply) + public_key = "ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQCzIeDhLeOVMfyYVV4ePCm/X4uRRmqkqq84TU2VsBDHWtMuFwBbez2ZmAm+WQ5zOyaZC/soSK17R8TsociZ+9wJBWT62aS3H8IHE2UxoakjlucF1QLM81oZaO5R4DCeKVJb0l/XfZ/fQkhYFLNM5622MbHP8MTTwfrbwE1+hjRFJYb0K364NCD0BLdgn+V7kfyEcSRRds8gh8zdiejJxFHRTaq9LRx+AQwFDkQYEYzk6ZGxIasKonCD18OtwAePdFgA1Mlho6Ajh9VyrgYWrEHKmfvDa/Rz7T/cCy5tkzdu5B04HWI7yBEthZeKm9QA8keOj1xU+yMWSNwUhiXGTg85 ec2-user@ip-10-0-0-44.us-west-2.compute.internal" } # module.networking.aws_default_route_table.tf_private_rt will be created + resource "aws_default_route_table" "tf_private_rt" { + default_route_table_id = (known after apply) + id = (known after apply) + owner_id = (known after apply) + route = (known after apply) + tags = { + "Name" = "tf_private" } + vpc_id = (known after apply) } # module.networking.aws_internet_gateway.tf_internet_gateway will be created + resource "aws_internet_gateway" "tf_internet_gateway" { + arn = (known after apply) + id = (known after apply) + owner_id = (known after apply) + tags = { + "Name" = "tf_igw" } + vpc_id = (known after apply) } # module.networking.aws_route_table.tf_public_rt will be created + resource "aws_route_table" "tf_public_rt" { + id = (known after apply) + owner_id = (known after apply) + propagating_vgws = (known after apply) + route = [ + { + cidr_block = "0.0.0.0/0" + egress_only_gateway_id = "" + gateway_id = (known after apply) + instance_id = "" + ipv6_cidr_block = "" + local_gateway_id = "" + nat_gateway_id = "" + network_interface_id = "" + transit_gateway_id = "" + vpc_peering_connection_id = "" }, ] + tags = { + "Name" = "tf_public" } + vpc_id = (known after apply) } # module.networking.aws_route_table_association.tf_public_assoc[0] will be created + resource "aws_route_table_association" "tf_public_assoc" { + id = (known after apply) + route_table_id = (known after apply) + subnet_id = (known after apply) } # module.networking.aws_route_table_association.tf_public_assoc[1] will be created + resource "aws_route_table_association" "tf_public_assoc" { + id = (known after apply) + route_table_id = (known after apply) + subnet_id = (known after apply) } # module.networking.aws_security_group.tf_public_sg will be created + resource "aws_security_group" "tf_public_sg" { + arn = (known after apply) + description = "Used for access to the public instances" + egress = [ + { + cidr_blocks = [ + "0.0.0.0/0", ] + description = "" + from_port = 0 + ipv6_cidr_blocks = [] + prefix_list_ids = [] + protocol = "-1" + security_groups = [] + self = false + to_port = 0 }, ] + id = (known after apply) + ingress = [ + { + cidr_blocks = [ + "0.0.0.0/0", ] + description = "" + from_port = 22 + ipv6_cidr_blocks = [] + prefix_list_ids = [] + protocol = "tcp" + security_groups = [] + self = false + to_port = 22 }, + { + cidr_blocks = [ + "0.0.0.0/0", ] + description = "" + from_port = 80 + ipv6_cidr_blocks = [] + prefix_list_ids = [] + protocol = "tcp" + security_groups = [] + self = false + to_port = 80 }, ] + name = "tf_public_sg" + owner_id = (known after apply) + revoke_rules_on_delete = false + vpc_id = (known after apply) } # module.networking.aws_subnet.tf_public_subnet[0] will be created + resource "aws_subnet" "tf_public_subnet" { + arn = (known after apply) + assign_ipv6_address_on_creation = false + availability_zone = "us-west-2a" + availability_zone_id = (known after apply) + cidr_block = "10.123.1.0/24" + id = (known after apply) + ipv6_cidr_block_association_id = (known after apply) + map_public_ip_on_launch = true + owner_id = (known after apply) + tags = { + "Name" = "tf_public_1" } + vpc_id = (known after apply) } # module.networking.aws_subnet.tf_public_subnet[1] will be created + resource "aws_subnet" "tf_public_subnet" { + arn = (known after apply) + assign_ipv6_address_on_creation = false + availability_zone = "us-west-2b" + availability_zone_id = (known after apply) + cidr_block = "10.123.2.0/24" + id = (known after apply) + ipv6_cidr_block_association_id = (known after apply) + map_public_ip_on_launch = true + owner_id = (known after apply) + tags = { + "Name" = "tf_public_2" } + vpc_id = (known after apply) } # module.networking.aws_vpc.tf_vpc will be created + resource "aws_vpc" "tf_vpc" { + arn = (known after apply) + assign_generated_ipv6_cidr_block = false + cidr_block = "10.123.0.0/16" + default_network_acl_id = (known after apply) + default_route_table_id = (known after apply) + default_security_group_id = (known after apply) + dhcp_options_id = (known after apply) + enable_classiclink = (known after apply) + enable_classiclink_dns_support = (known after apply) + enable_dns_hostnames = true + enable_dns_support = true + id = (known after apply) + instance_tenancy = "default" + ipv6_association_id = (known after apply) + ipv6_cidr_block = (known after apply) + main_route_table_id = (known after apply) + owner_id = (known after apply) + tags = { + "Name" = "tf_vpc" } } # module.storage.aws_s3_bucket.tf_code will be created + resource "aws_s3_bucket" "tf_code" { + acceleration_status = (known after apply) + acl = "private" + arn = (known after apply) + bucket = (known after apply) + bucket_domain_name = (known after apply) + bucket_regional_domain_name = (known after apply) + force_destroy = true + hosted_zone_id = (known after apply) + id = (known after apply) + region = (known after apply) + request_payer = (known after apply) + tags = { + "Name" = "tf_bucket" } + website_domain = (known after apply) + website_endpoint = (known after apply) + versioning { + enabled = (known after apply) + mfa_delete = (known after apply) } } # module.storage.random_id.tf_bucket_id will be created + resource "random_id" "tf_bucket_id" { + b64_std = (known after apply) + b64_url = (known after apply) + byte_length = 2 + dec = (known after apply) + hex = (known after apply) + id = (known after apply) } Plan: 14 to add, 0 to change, 0 to destroy. Changes to Outputs: + Bucket_Name = (known after apply) + Public_Instance_IDs = (known after apply) + Public_Instance_IPs = (known after apply) + Public_Security_Group = (known after apply) + Public_Subnets = (known after apply) + Subnet_IPs = "10.123.1.0/24, 10.123.2.0/24" Do you want to perform these actions? Terraform will perform the actions described above. Only 'yes' will be accepted to approve. Enter a value: yes module.storage.random_id.tf_bucket_id: Creating... module.storage.random_id.tf_bucket_id: Creation complete after 0s [id=ZtM] module.networking.aws_vpc.tf_vpc: Creating... module.compute.aws_key_pair.tf_auth: Creating... module.storage.aws_s3_bucket.tf_code: Creating... module.compute.aws_key_pair.tf_auth: Creation complete after 0s [id=tf_key] module.networking.aws_vpc.tf_vpc: Creation complete after 1s [id=vpc-091e518843d6ecb04] module.networking.aws_default_route_table.tf_private_rt: Creating... module.networking.aws_internet_gateway.tf_internet_gateway: Creating... module.networking.aws_subnet.tf_public_subnet[0]: Creating... module.networking.aws_security_group.tf_public_sg: Creating... module.networking.aws_subnet.tf_public_subnet[1]: Creating... module.networking.aws_default_route_table.tf_private_rt: Creation complete after 0s [id=rtb-07627bcc649fe3634] module.networking.aws_internet_gateway.tf_internet_gateway: Creation complete after 0s [id=igw-00b4b209a7da74eaf] module.networking.aws_route_table.tf_public_rt: Creating... module.storage.aws_s3_bucket.tf_code: Creation complete after 2s [id=la-terrafrom-26323] module.networking.aws_subnet.tf_public_subnet[0]: Creation complete after 1s [id=subnet-060302a46a656405b] module.networking.aws_subnet.tf_public_subnet[1]: Creation complete after 1s [id=subnet-0fbcea1253364514c] module.compute.data.template_file.user-init[0]: Reading... module.compute.data.template_file.user-init[0]: Read complete after 0s [id=57df141e108f0b6cf1703691b1a5a736ca3a01b0eb84e07196d778d405c36d19] module.compute.data.template_file.user-init[1]: Reading... module.compute.data.template_file.user-init[1]: Read complete after 0s [id=757bacc9f6377916bf38eff5b6acdb9ae2756c56859b9c203d8de26eff4865d4] module.networking.aws_route_table.tf_public_rt: Creation complete after 1s [id=rtb-0992d0e19d5dcbbe6] module.networking.aws_route_table_association.tf_public_assoc[1]: Creating... module.networking.aws_route_table_association.tf_public_assoc[0]: Creating... module.networking.aws_route_table_association.tf_public_assoc[0]: Creation complete after 0s [id=rtbassoc-0ea0e00e4ef1ff3df] module.networking.aws_route_table_association.tf_public_assoc[1]: Creation complete after 0s [id=rtbassoc-0d79338bd4c127b42] module.networking.aws_security_group.tf_public_sg: Creation complete after 1s [id=sg-094bcb58948a6a96d] module.compute.aws_instance.tf_server[0]: Creating... module.compute.aws_instance.tf_server[1]: Creating... module.compute.aws_instance.tf_server[0]: Still creating... [10s elapsed] module.compute.aws_instance.tf_server[1]: Still creating... [10s elapsed] module.compute.aws_instance.tf_server[0]: Still creating... [20s elapsed] module.compute.aws_instance.tf_server[1]: Still creating... [20s elapsed] module.compute.aws_instance.tf_server[1]: Creation complete after 21s [id=i-0186d81c4a1141e86] module.compute.aws_instance.tf_server[0]: Still creating... [30s elapsed] module.compute.aws_instance.tf_server[0]: Creation complete after 32s [id=i-02f7f327d22eb6dc6] Apply complete! Resources: 14 added, 0 changed, 0 destroyed. Outputs: Bucket_Name = la-terrafrom-26323 Public_Instance_IDs = i-02f7f327d22eb6dc6, i-0186d81c4a1141e86 Public_Instance_IPs = 54.214.69.170, 35.166.52.155 Public_Security_Group = sg-094bcb58948a6a96d Public_Subnets = subnet-060302a46a656405b, subnet-0fbcea1253364514c Subnet_IPs = 10.123.1.0/24, 10.123.2.0/24
Object terraform.tfstate appeared under prefix terraform of S3 bucket la-terraform-course-state-sc.

ec2-user:~/environment/AWS $ ls
compute networking storage terraform.tfstate.backup variables.tf main.tf outputs.tf terraform.tfstate terraform.tfvars
ec2-user:~/environment/AWS $ terraform show
# module.compute.aws_instance.tf_server[0]: resource "aws_instance" "tf_server" { ami = "ami-01fee56b22f308154" arn = "arn:aws:ec2:us-west-2:124011853020:instance/i-02f7f327d22eb6dc6" associate_public_ip_address = true availability_zone = "us-west-2a" cpu_core_count = 1 cpu_threads_per_core = 1 disable_api_termination = false ebs_optimized = false get_password_data = false hibernation = false id = "i-02f7f327d22eb6dc6" instance_state = "running" instance_type = "t2.micro" ipv6_address_count = 0 ipv6_addresses = [] key_name = "tf_key" monitoring = false primary_network_interface_id = "eni-05c1941948113009b" private_dns = "ip-10-123-1-150.us-west-2.compute.internal" private_ip = "10.123.1.150" public_dns = "ec2-54-214-69-170.us-west-2.compute.amazonaws.com" public_ip = "54.214.69.170" secondary_private_ips = [] security_groups = [] source_dest_check = true subnet_id = "subnet-060302a46a656405b" tags = { "Name" = "tf_server-1" } tenancy = "default" user_data = "544105fc76f56380fa17cd0686e033cee5001d87" volume_tags = {} vpc_security_group_ids = [ "sg-094bcb58948a6a96d", ] credit_specification { cpu_credits = "standard" } metadata_options { http_endpoint = "enabled" http_put_response_hop_limit = 1 http_tokens = "optional" } root_block_device { delete_on_termination = true device_name = "/dev/xvda" encrypted = false iops = 100 volume_id = "vol-0ed8336a4bd536539" volume_size = 8 volume_type = "gp2" } } # module.compute.aws_instance.tf_server[1]: resource "aws_instance" "tf_server" { ami = "ami-01fee56b22f308154" arn = "arn:aws:ec2:us-west-2:124011853020:instance/i-0186d81c4a1141e86" associate_public_ip_address = true availability_zone = "us-west-2b" cpu_core_count = 1 cpu_threads_per_core = 1 disable_api_termination = false ebs_optimized = false get_password_data = false hibernation = false id = "i-0186d81c4a1141e86" instance_state = "running" instance_type = "t2.micro" ipv6_address_count = 0 ipv6_addresses = [] key_name = "tf_key" monitoring = false primary_network_interface_id = "eni-05cd8904109496549" private_dns = "ip-10-123-2-95.us-west-2.compute.internal" private_ip = "10.123.2.95" public_dns = "ec2-35-166-52-155.us-west-2.compute.amazonaws.com" public_ip = "35.166.52.155" secondary_private_ips = [] security_groups = [] source_dest_check = true subnet_id = "subnet-0fbcea1253364514c" tags = { "Name" = "tf_server-2" } tenancy = "default" user_data = "ea5b38a77b74322af7f46802b7e74b3277c7eb0d" volume_tags = {} vpc_security_group_ids = [ "sg-094bcb58948a6a96d", ] credit_specification { cpu_credits = "standard" } metadata_options { http_endpoint = "enabled" http_put_response_hop_limit = 1 http_tokens = "optional" } root_block_device { delete_on_termination = true device_name = "/dev/xvda" encrypted = false iops = 100 volume_id = "vol-03211f99c46070a7c" volume_size = 8 volume_type = "gp2" } } # module.compute.aws_key_pair.tf_auth: resource "aws_key_pair" "tf_auth" { arn = "arn:aws:ec2:us-west-2:124011853020:key-pair/tf_key" fingerprint = "9d:54:ea:2a:70:a8:52:d7:4c:56:16:0f:8b:83:2e:3b" id = "tf_key" key_name = "tf_key" key_pair_id = "key-0fe18333b70817847" public_key = "ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQCzIeDhLeOVMfyYVV4ePCm/X4uRRmqkqq84TU2VsBDHWtMuFwBbez2ZmAm+WQ5zOyaZC/soSK17R8TsociZ+9wJBWT62aS3H8IHE2UxoakjlucF1QLM81oZaO5R4DCeKVJb0l/XfZ/fQkhYFLNM5622MbHP8MTTwfrbwE1+hjRFJYb0K364NCD0BLdgn+V7kfyEcSRRds8gh8zdiejJxFHRTaq9LRx+AQwFDkQYEYzk6ZGxIasKonCD18OtwAePdFgA1Mlho6Ajh9VyrgYWrEHKmfvDa/Rz7T/cCy5tkzdu5B04HWI7yBEthZeKm9QA8keOj1xU+yMWSNwUhiXGTg85 ec2-user@ip-10-0-0-44.us-west-2.compute.internal" } # module.compute.data.aws_ami.server_ami: data "aws_ami" "server_ami" { architecture = "x86_64" arn = "arn:aws:ec2:us-west-2::image/ami-01fee56b22f308154" block_device_mappings = [ { device_name = "/dev/xvda" ebs = { "delete_on_termination" = "true" "encrypted" = "false" "iops" = "0" "snapshot_id" = "snap-0ab50542cd4337d4f" "volume_size" = "8" "volume_type" = "gp2" } no_device = "" virtual_name = "" }, ] creation_date = "2020-09-23T17:37:54.000Z" description = "Amazon Linux AMI 2018.03.0.20200918.0 x86_64 HVM gp2" hypervisor = "xen" id = "ami-01fee56b22f308154" image_id = "ami-01fee56b22f308154" image_location = "amazon/amzn-ami-hvm-2018.03.0.20200918.0-x86_64-gp2" image_owner_alias = "amazon" image_type = "machine" most_recent = true name = "amzn-ami-hvm-2018.03.0.20200918.0-x86_64-gp2" owner_id = "137112412989" owners = [ "amazon", ] product_codes = [] public = true root_device_name = "/dev/xvda" root_device_type = "ebs" root_snapshot_id = "snap-0ab50542cd4337d4f" sriov_net_support = "simple" state = "available" state_reason = { "code" = "UNSET" "message" = "UNSET" } tags = {} virtualization_type = "hvm" filter { name = "name" values = [ "amzn-ami-hvm*-x86_64-gp2", ] } filter { name = "owner-alias" values = [ "amazon", ] } } # module.compute.data.template_file.user-init[0]: data "template_file" "user-init" { id = "57df141e108f0b6cf1703691b1a5a736ca3a01b0eb84e07196d778d405c36d19" rendered = <<~EOT #!/bin/bash yum install httpd -y echo "Subnet for Firewall: 10.123.1.0/24" >> /var/www/html/index.html service httpd start chkconfig httpd on EOT template = <<~EOT #!/bin/bash yum install httpd -y echo "Subnet for Firewall: ${firewall_subnets}" >> /var/www/html/index.html service httpd start chkconfig httpd on EOT vars = { "firewall_subnets" = "10.123.1.0/24" } } # module.compute.data.template_file.user-init[1]: data "template_file" "user-init" { id = "757bacc9f6377916bf38eff5b6acdb9ae2756c56859b9c203d8de26eff4865d4" rendered = <<~EOT #!/bin/bash yum install httpd -y echo "Subnet for Firewall: 10.123.2.0/24" >> /var/www/html/index.html service httpd start chkconfig httpd on EOT template = <<~EOT #!/bin/bash yum install httpd -y echo "Subnet for Firewall: ${firewall_subnets}" >> /var/www/html/index.html service httpd start chkconfig httpd on EOT vars = { "firewall_subnets" = "10.123.2.0/24" } } # module.networking.aws_default_route_table.tf_private_rt: resource "aws_default_route_table" "tf_private_rt" { default_route_table_id = "rtb-07627bcc649fe3634" id = "rtb-07627bcc649fe3634" owner_id = "124011853020" route = [] tags = { "Name" = "tf_private" } vpc_id = "vpc-091e518843d6ecb04" } # module.networking.aws_internet_gateway.tf_internet_gateway: resource "aws_internet_gateway" "tf_internet_gateway" { arn = "arn:aws:ec2:us-west-2:124011853020:internet-gateway/igw-00b4b209a7da74eaf" id = "igw-00b4b209a7da74eaf" owner_id = "124011853020" tags = { "Name" = "tf_igw" } vpc_id = "vpc-091e518843d6ecb04" } # module.networking.aws_route_table.tf_public_rt: resource "aws_route_table" "tf_public_rt" { id = "rtb-0992d0e19d5dcbbe6" owner_id = "124011853020" propagating_vgws = [] route = [ { cidr_block = "0.0.0.0/0" egress_only_gateway_id = "" gateway_id = "igw-00b4b209a7da74eaf" instance_id = "" ipv6_cidr_block = "" local_gateway_id = "" nat_gateway_id = "" network_interface_id = "" transit_gateway_id = "" vpc_peering_connection_id = "" }, ] tags = { "Name" = "tf_public" } vpc_id = "vpc-091e518843d6ecb04" } # module.networking.aws_route_table_association.tf_public_assoc[1]: resource "aws_route_table_association" "tf_public_assoc" { id = "rtbassoc-0d79338bd4c127b42" route_table_id = "rtb-0992d0e19d5dcbbe6" subnet_id = "subnet-0fbcea1253364514c" } # module.networking.aws_route_table_association.tf_public_assoc[0]: resource "aws_route_table_association" "tf_public_assoc" { id = "rtbassoc-0ea0e00e4ef1ff3df" route_table_id = "rtb-0992d0e19d5dcbbe6" subnet_id = "subnet-060302a46a656405b" } # module.networking.aws_security_group.tf_public_sg: resource "aws_security_group" "tf_public_sg" { arn = "arn:aws:ec2:us-west-2:124011853020:security-group/sg-094bcb58948a6a96d" description = "Used for access to the public instances" egress = [ { cidr_blocks = [ "0.0.0.0/0", ] description = "" from_port = 0 ipv6_cidr_blocks = [] prefix_list_ids = [] protocol = "-1" security_groups = [] self = false to_port = 0 }, ] id = "sg-094bcb58948a6a96d" ingress = [ { cidr_blocks = [ "0.0.0.0/0", ] description = "" from_port = 22 ipv6_cidr_blocks = [] prefix_list_ids = [] protocol = "tcp" security_groups = [] self = false to_port = 22 }, { cidr_blocks = [ "0.0.0.0/0", ] description = "" from_port = 80 ipv6_cidr_blocks = [] prefix_list_ids = [] protocol = "tcp" security_groups = [] self = false to_port = 80 }, ] name = "tf_public_sg" owner_id = "124011853020" revoke_rules_on_delete = false vpc_id = "vpc-091e518843d6ecb04" } # module.networking.aws_subnet.tf_public_subnet[0]: resource "aws_subnet" "tf_public_subnet" { arn = "arn:aws:ec2:us-west-2:124011853020:subnet/subnet-060302a46a656405b" assign_ipv6_address_on_creation = false availability_zone = "us-west-2a" availability_zone_id = "usw2-az1" cidr_block = "10.123.1.0/24" id = "subnet-060302a46a656405b" map_public_ip_on_launch = true owner_id = "124011853020" tags = { "Name" = "tf_public_1" } vpc_id = "vpc-091e518843d6ecb04" } # module.networking.aws_subnet.tf_public_subnet[1]: resource "aws_subnet" "tf_public_subnet" { arn = "arn:aws:ec2:us-west-2:124011853020:subnet/subnet-0fbcea1253364514c" assign_ipv6_address_on_creation = false availability_zone = "us-west-2b" availability_zone_id = "usw2-az2" cidr_block = "10.123.2.0/24" id = "subnet-0fbcea1253364514c" map_public_ip_on_launch = true owner_id = "124011853020" tags = { "Name" = "tf_public_2" } vpc_id = "vpc-091e518843d6ecb04" } # module.networking.aws_vpc.tf_vpc: resource "aws_vpc" "tf_vpc" { arn = "arn:aws:ec2:us-west-2:124011853020:vpc/vpc-091e518843d6ecb04" assign_generated_ipv6_cidr_block = false cidr_block = "10.123.0.0/16" default_network_acl_id = "acl-0cdb8cb004bdfa1ec" default_route_table_id = "rtb-07627bcc649fe3634" default_security_group_id = "sg-022aeddefab97e010" dhcp_options_id = "dopt-cc5342a9" enable_classiclink = false enable_classiclink_dns_support = false enable_dns_hostnames = true enable_dns_support = true id = "vpc-091e518843d6ecb04" instance_tenancy = "default" main_route_table_id = "rtb-07627bcc649fe3634" owner_id = "124011853020" tags = { "Name" = "tf_vpc" } } # module.networking.data.aws_availability_zones.available: data "aws_availability_zones" "available" { group_names = [ "us-west-2", ] id = "2020-10-10 01:49:21.78689905 +0000 UTC" names = [ "us-west-2a", "us-west-2b", "us-west-2c", "us-west-2d", ] zone_ids = [ "usw2-az1", "usw2-az2", "usw2-az3", "usw2-az4", ] } # module.storage.aws_s3_bucket.tf_code: resource "aws_s3_bucket" "tf_code" { acl = "private" arn = "arn:aws:s3:::la-terrafrom-26323" bucket = "la-terrafrom-26323" bucket_domain_name = "la-terrafrom-26323.s3.amazonaws.com" bucket_regional_domain_name = "la-terrafrom-26323.s3.us-west-2.amazonaws.com" force_destroy = true hosted_zone_id = "Z3BJ6K6RIION7M" id = "la-terrafrom-26323" region = "us-west-2" request_payer = "BucketOwner" tags = { "Name" = "tf_bucket" } versioning { enabled = false mfa_delete = false } } # module.storage.random_id.tf_bucket_id: resource "random_id" "tf_bucket_id" { b64_std = "ZtM=" b64_url = "ZtM" byte_length = 2 dec = "26323" hex = "66d3" id = "ZtM" } Outputs: Bucket_Name = "la-terrafrom-26323" Public_Instance_IDs = "i-02f7f327d22eb6dc6, i-0186d81c4a1141e86" Public_Instance_IPs = "54.214.69.170, 35.166.52.155" Public_Security_Group = "sg-094bcb58948a6a96d" Public_Subnets = "subnet-060302a46a656405b, subnet-0fbcea1253364514c" Subnet_IPs = "10.123.1.0/24, 10.123.2.0/24"
ec2-user:~/environment/AWS $ mv terraform.tfstate terraform.tfstate.bak
ec2-user:~/environment/AWS $ terraform show
# module.storage.aws_s3_bucket.tf_code: resource "aws_s3_bucket" "tf_code" { acl = "private" arn = "arn:aws:s3:::la-terrafrom-26323" bucket = "la-terrafrom-26323" bucket_domain_name = "la-terrafrom-26323.s3.amazonaws.com" bucket_regional_domain_name = "la-terrafrom-26323.s3.us-west-2.amazonaws.com" force_destroy = true hosted_zone_id = "Z3BJ6K6RIION7M" id = "la-terrafrom-26323" region = "us-west-2" request_payer = "BucketOwner" tags = { "Name" = "tf_bucket" } versioning { enabled = false mfa_delete = false } } # module.storage.random_id.tf_bucket_id: resource "random_id" "tf_bucket_id" { b64_std = "ZtM=" b64_url = "ZtM" byte_length = 2 dec = "26323" hex = "66d3" id = "ZtM" } # module.compute.aws_instance.tf_server[0]: resource "aws_instance" "tf_server" { ami = "ami-01fee56b22f308154" arn = "arn:aws:ec2:us-west-2:124011853020:instance/i-02f7f327d22eb6dc6" associate_public_ip_address = true availability_zone = "us-west-2a" cpu_core_count = 1 cpu_threads_per_core = 1 disable_api_termination = false ebs_optimized = false get_password_data = false hibernation = false id = "i-02f7f327d22eb6dc6" instance_state = "running" instance_type = "t2.micro" ipv6_address_count = 0 ipv6_addresses = [] key_name = "tf_key" monitoring = false primary_network_interface_id = "eni-05c1941948113009b" private_dns = "ip-10-123-1-150.us-west-2.compute.internal" private_ip = "10.123.1.150" public_dns = "ec2-54-214-69-170.us-west-2.compute.amazonaws.com" public_ip = "54.214.69.170" secondary_private_ips = [] security_groups = [] source_dest_check = true subnet_id = "subnet-060302a46a656405b" tags = { "Name" = "tf_server-1" } tenancy = "default" user_data = "544105fc76f56380fa17cd0686e033cee5001d87" volume_tags = {} vpc_security_group_ids = [ "sg-094bcb58948a6a96d", ] credit_specification { cpu_credits = "standard" } metadata_options { http_endpoint = "enabled" http_put_response_hop_limit = 1 http_tokens = "optional" } root_block_device { delete_on_termination = true device_name = "/dev/xvda" encrypted = false iops = 100 volume_id = "vol-0ed8336a4bd536539" volume_size = 8 volume_type = "gp2" } } # module.compute.aws_instance.tf_server[1]: resource "aws_instance" "tf_server" { ami = "ami-01fee56b22f308154" arn = "arn:aws:ec2:us-west-2:124011853020:instance/i-0186d81c4a1141e86" associate_public_ip_address = true availability_zone = "us-west-2b" cpu_core_count = 1 cpu_threads_per_core = 1 disable_api_termination = false ebs_optimized = false get_password_data = false hibernation = false id = "i-0186d81c4a1141e86" instance_state = "running" instance_type = "t2.micro" ipv6_address_count = 0 ipv6_addresses = [] key_name = "tf_key" monitoring = false primary_network_interface_id = "eni-05cd8904109496549" private_dns = "ip-10-123-2-95.us-west-2.compute.internal" private_ip = "10.123.2.95" public_dns = "ec2-35-166-52-155.us-west-2.compute.amazonaws.com" public_ip = "35.166.52.155" secondary_private_ips = [] security_groups = [] source_dest_check = true subnet_id = "subnet-0fbcea1253364514c" tags = { "Name" = "tf_server-2" } tenancy = "default" user_data = "ea5b38a77b74322af7f46802b7e74b3277c7eb0d" volume_tags = {} vpc_security_group_ids = [ "sg-094bcb58948a6a96d", ] credit_specification { cpu_credits = "standard" } metadata_options { http_endpoint = "enabled" http_put_response_hop_limit = 1 http_tokens = "optional" } root_block_device { delete_on_termination = true device_name = "/dev/xvda" encrypted = false iops = 100 volume_id = "vol-03211f99c46070a7c" volume_size = 8 volume_type = "gp2" } } # module.compute.aws_key_pair.tf_auth: resource "aws_key_pair" "tf_auth" { arn = "arn:aws:ec2:us-west-2:124011853020:key-pair/tf_key" fingerprint = "9d:54:ea:2a:70:a8:52:d7:4c:56:16:0f:8b:83:2e:3b" id = "tf_key" key_name = "tf_key" key_pair_id = "key-0fe18333b70817847" public_key = "ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQCzIeDhLeOVMfyYVV4ePCm/X4uRRmqkqq84TU2VsBDHWtMuFwBbez2ZmAm+WQ5zOyaZC/soSK17R8TsociZ+9wJBWT62aS3H8IHE2UxoakjlucF1QLM81oZaO5R4DCeKVJb0l/XfZ/fQkhYFLNM5622MbHP8MTTwfrbwE1+hjRFJYb0K364NCD0BLdgn+V7kfyEcSRRds8gh8zdiejJxFHRTaq9LRx+AQwFDkQYEYzk6ZGxIasKonCD18OtwAePdFgA1Mlho6Ajh9VyrgYWrEHKmfvDa/Rz7T/cCy5tkzdu5B04HWI7yBEthZeKm9QA8keOj1xU+yMWSNwUhiXGTg85 ec2-user@ip-10-0-0-44.us-west-2.compute.internal" } # module.compute.data.aws_ami.server_ami: data "aws_ami" "server_ami" { architecture = "x86_64" arn = "arn:aws:ec2:us-west-2::image/ami-01fee56b22f308154" block_device_mappings = [ { device_name = "/dev/xvda" ebs = { "delete_on_termination" = "true" "encrypted" = "false" "iops" = "0" "snapshot_id" = "snap-0ab50542cd4337d4f" "volume_size" = "8" "volume_type" = "gp2" } no_device = "" virtual_name = "" }, ] creation_date = "2020-09-23T17:37:54.000Z" description = "Amazon Linux AMI 2018.03.0.20200918.0 x86_64 HVM gp2" hypervisor = "xen" id = "ami-01fee56b22f308154" image_id = "ami-01fee56b22f308154" image_location = "amazon/amzn-ami-hvm-2018.03.0.20200918.0-x86_64-gp2" image_owner_alias = "amazon" image_type = "machine" most_recent = true name = "amzn-ami-hvm-2018.03.0.20200918.0-x86_64-gp2" owner_id = "137112412989" owners = [ "amazon", ] product_codes = [] public = true root_device_name = "/dev/xvda" root_device_type = "ebs" root_snapshot_id = "snap-0ab50542cd4337d4f" sriov_net_support = "simple" state = "available" state_reason = { "code" = "UNSET" "message" = "UNSET" } tags = {} virtualization_type = "hvm" filter { name = "name" values = [ "amzn-ami-hvm*-x86_64-gp2", ] } filter { name = "owner-alias" values = [ "amazon", ] } } # module.compute.data.template_file.user-init[0]: data "template_file" "user-init" { id = "57df141e108f0b6cf1703691b1a5a736ca3a01b0eb84e07196d778d405c36d19" rendered = <<~EOT #!/bin/bash yum install httpd -y echo "Subnet for Firewall: 10.123.1.0/24" >> /var/www/html/index.html service httpd start chkconfig httpd on EOT template = <<~EOT #!/bin/bash yum install httpd -y echo "Subnet for Firewall: ${firewall_subnets}" >> /var/www/html/index.html service httpd start chkconfig httpd on EOT vars = { "firewall_subnets" = "10.123.1.0/24" } } # module.compute.data.template_file.user-init[1]: data "template_file" "user-init" { id = "757bacc9f6377916bf38eff5b6acdb9ae2756c56859b9c203d8de26eff4865d4" rendered = <<~EOT #!/bin/bash yum install httpd -y echo "Subnet for Firewall: 10.123.2.0/24" >> /var/www/html/index.html service httpd start chkconfig httpd on EOT template = <<~EOT #!/bin/bash yum install httpd -y echo "Subnet for Firewall: ${firewall_subnets}" >> /var/www/html/index.html service httpd start chkconfig httpd on EOT vars = { "firewall_subnets" = "10.123.2.0/24" } } # module.networking.aws_default_route_table.tf_private_rt: resource "aws_default_route_table" "tf_private_rt" { default_route_table_id = "rtb-07627bcc649fe3634" id = "rtb-07627bcc649fe3634" owner_id = "124011853020" route = [] tags = { "Name" = "tf_private" } vpc_id = "vpc-091e518843d6ecb04" } # module.networking.aws_internet_gateway.tf_internet_gateway: resource "aws_internet_gateway" "tf_internet_gateway" { arn = "arn:aws:ec2:us-west-2:124011853020:internet-gateway/igw-00b4b209a7da74eaf" id = "igw-00b4b209a7da74eaf" owner_id = "124011853020" tags = { "Name" = "tf_igw" } vpc_id = "vpc-091e518843d6ecb04" } # module.networking.aws_route_table.tf_public_rt: resource "aws_route_table" "tf_public_rt" { id = "rtb-0992d0e19d5dcbbe6" owner_id = "124011853020" propagating_vgws = [] route = [ { cidr_block = "0.0.0.0/0" egress_only_gateway_id = "" gateway_id = "igw-00b4b209a7da74eaf" instance_id = "" ipv6_cidr_block = "" local_gateway_id = "" nat_gateway_id = "" network_interface_id = "" transit_gateway_id = "" vpc_peering_connection_id = "" }, ] tags = { "Name" = "tf_public" } vpc_id = "vpc-091e518843d6ecb04" } # module.networking.aws_route_table_association.tf_public_assoc[1]: resource "aws_route_table_association" "tf_public_assoc" { id = "rtbassoc-0d79338bd4c127b42" route_table_id = "rtb-0992d0e19d5dcbbe6" subnet_id = "subnet-0fbcea1253364514c" } # module.networking.aws_route_table_association.tf_public_assoc[0]: resource "aws_route_table_association" "tf_public_assoc" { id = "rtbassoc-0ea0e00e4ef1ff3df" route_table_id = "rtb-0992d0e19d5dcbbe6" subnet_id = "subnet-060302a46a656405b" } # module.networking.aws_security_group.tf_public_sg: resource "aws_security_group" "tf_public_sg" { arn = "arn:aws:ec2:us-west-2:124011853020:security-group/sg-094bcb58948a6a96d" description = "Used for access to the public instances" egress = [ { cidr_blocks = [ "0.0.0.0/0", ] description = "" from_port = 0 ipv6_cidr_blocks = [] prefix_list_ids = [] protocol = "-1" security_groups = [] self = false to_port = 0 }, ] id = "sg-094bcb58948a6a96d" ingress = [ { cidr_blocks = [ "0.0.0.0/0", ] description = "" from_port = 22 ipv6_cidr_blocks = [] prefix_list_ids = [] protocol = "tcp" security_groups = [] self = false to_port = 22 }, { cidr_blocks = [ "0.0.0.0/0", ] description = "" from_port = 80 ipv6_cidr_blocks = [] prefix_list_ids = [] protocol = "tcp" security_groups = [] self = false to_port = 80 }, ] name = "tf_public_sg" owner_id = "124011853020" revoke_rules_on_delete = false vpc_id = "vpc-091e518843d6ecb04" } # module.networking.aws_subnet.tf_public_subnet[1]: resource "aws_subnet" "tf_public_subnet" { arn = "arn:aws:ec2:us-west-2:124011853020:subnet/subnet-0fbcea1253364514c" assign_ipv6_address_on_creation = false availability_zone = "us-west-2b" availability_zone_id = "usw2-az2" cidr_block = "10.123.2.0/24" id = "subnet-0fbcea1253364514c" map_public_ip_on_launch = true owner_id = "124011853020" tags = { "Name" = "tf_public_2" } vpc_id = "vpc-091e518843d6ecb04" } # module.networking.aws_subnet.tf_public_subnet[0]: resource "aws_subnet" "tf_public_subnet" { arn = "arn:aws:ec2:us-west-2:124011853020:subnet/subnet-060302a46a656405b" assign_ipv6_address_on_creation = false availability_zone = "us-west-2a" availability_zone_id = "usw2-az1" cidr_block = "10.123.1.0/24" id = "subnet-060302a46a656405b" map_public_ip_on_launch = true owner_id = "124011853020" tags = { "Name" = "tf_public_1" } vpc_id = "vpc-091e518843d6ecb04" } # module.networking.aws_vpc.tf_vpc: resource "aws_vpc" "tf_vpc" { arn = "arn:aws:ec2:us-west-2:124011853020:vpc/vpc-091e518843d6ecb04" assign_generated_ipv6_cidr_block = false cidr_block = "10.123.0.0/16" default_network_acl_id = "acl-0cdb8cb004bdfa1ec" default_route_table_id = "rtb-07627bcc649fe3634" default_security_group_id = "sg-022aeddefab97e010" dhcp_options_id = "dopt-cc5342a9" enable_classiclink = false enable_classiclink_dns_support = false enable_dns_hostnames = true enable_dns_support = true id = "vpc-091e518843d6ecb04" instance_tenancy = "default" main_route_table_id = "rtb-07627bcc649fe3634" owner_id = "124011853020" tags = { "Name" = "tf_vpc" } } # module.networking.data.aws_availability_zones.available: data "aws_availability_zones" "available" { group_names = [ "us-west-2", ] id = "2020-10-10 01:49:21.78689905 +0000 UTC" names = [ "us-west-2a", "us-west-2b", "us-west-2c", "us-west-2d", ] zone_ids = [ "usw2-az1", "usw2-az2", "usw2-az3", "usw2-az4", ] } Outputs: Bucket_Name = "la-terrafrom-26323" Public_Instance_IDs = "i-02f7f327d22eb6dc6, i-0186d81c4a1141e86" Public_Instance_IPs = "54.214.69.170, 35.166.52.155" Public_Security_Group = "sg-094bcb58948a6a96d" Public_Subnets = "subnet-060302a46a656405b, subnet-0fbcea1253364514c" Subnet_IPs = "10.123.1.0/24, 10.123.2.0/24"
Rename object terraform.tfstate to terraform.tfstate.bak
ec2-user:~/environment/AWS $ terraform show
No state.
*
ec2-user:~/environment/AWS $ mv terraform.tfstate.bak terraform.tfstate
ec2-user:~/environment/AWS $ terraform show
No state.
*
Rename object terraform.tfstate.bak back to terraform.tfstate
ec2-user:~/environment/AWS $ terraform show
module.compute.aws_instance.tf_server.0: id = i-0c881f15e939d1bb6 ami = ami-a0cfeed8 arn = arn:aws:ec2:us-west-2:124011853020:instance/i-0c881f15e939d1bb6 associate_public_ip_address = true availability_zone = us-west-2a cpu_core_count = 1 cpu_threads_per_core = 1 credit_specification.# = 1 credit_specification.0.cpu_credits = standard disable_api_termination = false ebs_block_device.# = 0 ebs_optimized = false ephemeral_block_device.# = 0 get_password_data = false iam_instance_profile = instance_state = running instance_type = t2.micro ipv6_addresses.# = 0 key_name = tf_key monitoring = false network_interface.# = 0 network_interface_id = eni-0d53c4bd75a6912c1 password_data = placement_group = primary_network_interface_id = eni-0d53c4bd75a6912c1 private_dns = ip-10-123-1-244.us-west-2.compute.internal private_ip = 10.123.1.244 public_dns = ec2-34-215-49-88.us-west-2.compute.amazonaws.com public_ip = 34.215.49.88 root_block_device.# = 1 root_block_device.0.delete_on_termination = true root_block_device.0.iops = 100 root_block_device.0.volume_id = vol-0440e45e90914c4af root_block_device.0.volume_size = 8 root_block_device.0.volume_type = gp2 security_groups.# = 0 source_dest_check = true subnet_id = subnet-0e6566095894ef715 tags.% = 1 tags.Name = tf_server-1 tenancy = default user_data = 544105fc76f56380fa17cd0686e033cee5001d87 volume_tags.% = 0 vpc_security_group_ids.# = 1 vpc_security_group_ids.1328413595 = sg-0c7ed456b9ed183c9 module.compute.aws_instance.tf_server.1: id = i-00a1b4e02788b49e9 ami = ami-a0cfeed8 arn = arn:aws:ec2:us-west-2:124011853020:instance/i-00a1b4e02788b49e9 associate_public_ip_address = true availability_zone = us-west-2b cpu_core_count = 1 cpu_threads_per_core = 1 credit_specification.# = 1 credit_specification.0.cpu_credits = standard disable_api_termination = false ebs_block_device.# = 0 ebs_optimized = false ephemeral_block_device.# = 0 get_password_data = false iam_instance_profile = instance_state = running instance_type = t2.micro ipv6_addresses.# = 0 key_name = tf_key monitoring = false network_interface.# = 0 network_interface_id = eni-0480b88b083468b0d password_data = placement_group = primary_network_interface_id = eni-0480b88b083468b0d private_dns = ip-10-123-2-131.us-west-2.compute.internal private_ip = 10.123.2.131 public_dns = ec2-34-214-144-245.us-west-2.compute.amazonaws.com public_ip = 34.214.144.245 root_block_device.# = 1 root_block_device.0.delete_on_termination = true root_block_device.0.iops = 100 root_block_device.0.volume_id = vol-0ab29eb178158c5ae root_block_device.0.volume_size = 8 root_block_device.0.volume_type = gp2 security_groups.# = 0 source_dest_check = true subnet_id = subnet-0cca01a76090c47be tags.% = 1 tags.Name = tf_server-2 tenancy = default user_data = ea5b38a77b74322af7f46802b7e74b3277c7eb0d volume_tags.% = 0 vpc_security_group_ids.# = 1 vpc_security_group_ids.1328413595 = sg-0c7ed456b9ed183c9 module.compute.aws_key_pair.tf_auth: id = tf_key fingerprint = ed:16:51:7d:6a:9b:6c:46:eb:1d:2f:69:c4:9a:ad:fe key_name = tf_key public_key = ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQCnYE+D6epfc7eo5whpIlD6NS5tzVYJqm1RRWZoG2aloZO2AAPiSDQfQLoEOLv1DfHNvKBtckDR2ifyEb3thg/Ect+PNmk04qT9a6yGj4T5P+TW4y8P5ha4It3NyauNLXMKuzVu9M3dUOQbbFfnwGf8cE0pFqcw9bVlvhM5Lo68anyaTyH6+fwLCS0Q+sSFpmwY5LoGO0LknPyltcFmXU4xiX/gkT6+3KYE3JDKtDqNbskslemjfxDx+X3lzcbWVw1UayB69cKFS4MFkf2EVkXnCqSz9V2lks3270YJzbte/rtIUwbG+JRTDcqpsi1/zI2iOxOqdx+BER7bGpVc+WOZ ec2-user@ip-172-31-34-62 module.compute.data.aws_ami.server_ami: id = ami-a0cfeed8 architecture = x86_64 block_device_mappings.# = 1 block_device_mappings.340275815.device_name = /dev/xvda block_device_mappings.340275815.ebs.% = 6 block_device_mappings.340275815.ebs.delete_on_termination = true block_device_mappings.340275815.ebs.encrypted = false block_device_mappings.340275815.ebs.iops = 0 block_device_mappings.340275815.ebs.snapshot_id = snap-0b9ac5da0147e5eb2 block_device_mappings.340275815.ebs.volume_size = 8 block_device_mappings.340275815.ebs.volume_type = gp2 block_device_mappings.340275815.no_device = block_device_mappings.340275815.virtual_name = creation_date = 2018-08-11T02:29:45.000Z description = Amazon Linux AMI 2018.03.0.20180811 x86_64 HVM GP2 filter.# = 2 filter.2898439165.name = name filter.2898439165.values.# = 1 filter.2898439165.values.0 = amzn-ami-hvm*-x86_64-gp2 filter.574890044.name = owner-alias filter.574890044.values.# = 1 filter.574890044.values.0 = amazon hypervisor = xen image_id = ami-a0cfeed8 image_location = amazon/amzn-ami-hvm-2018.03.0.20180811-x86_64-gp2 image_owner_alias = amazon image_type = machine most_recent = true name = amzn-ami-hvm-2018.03.0.20180811-x86_64-gp2 owner_id = 137112412989 product_codes.# = 0 public = true root_device_name = /dev/xvda root_device_type = ebs root_snapshot_id = snap-0b9ac5da0147e5eb2 sriov_net_support = simple state = available state_reason.% = 2 state_reason.code = UNSET state_reason.message = UNSET tags.% = 0 virtualization_type = hvm module.compute.data.template_file.user-init.0: id = 57df141e108f0b6cf1703691b1a5a736ca3a01b0eb84e07196d778d405c36d19 rendered = #!/bin/bash yum install httpd -y echo "Subnet for Firewall: 10.123.1.0/24" >> /var/www/html/index.html service httpd start chkconfig httpd on template = #!/bin/bash yum install httpd -y echo "Subnet for Firewall: ${firewall_subnets}" >> /var/www/html/index.html service httpd start chkconfig httpd on vars.% = 1 vars.firewall_subnets = 10.123.1.0/24 module.compute.data.template_file.user-init.1: id = 757bacc9f6377916bf38eff5b6acdb9ae2756c56859b9c203d8de26eff4865d4 rendered = #!/bin/bash yum install httpd -y echo "Subnet for Firewall: 10.123.2.0/24" >> /var/www/html/index.html service httpd start chkconfig httpd on template = #!/bin/bash yum install httpd -y echo "Subnet for Firewall: ${firewall_subnets}" >> /var/www/html/index.html service httpd start chkconfig httpd on vars.% = 1 vars.firewall_subnets = 10.123.2.0/24 module.networking.aws_internet_gateway.tf_internet_gateway: id = igw-0f029a225e9bb81f9 tags.% = 1 tags.Name = tf_igw vpc_id = vpc-05498c93b1d9a8ab3 module.networking.aws_route_table.tf_public_rt: id = rtb-079cac14ba01dbfc5 propagating_vgws.# = 0 route.# = 1 route.2962636803.cidr_block = 0.0.0.0/0 route.2962636803.egress_only_gateway_id = route.2962636803.gateway_id = igw-0f029a225e9bb81f9 route.2962636803.instance_id = route.2962636803.ipv6_cidr_block = route.2962636803.nat_gateway_id = route.2962636803.network_interface_id = route.2962636803.vpc_peering_connection_id = tags.% = 1 tags.Name = tf_public vpc_id = vpc-05498c93b1d9a8ab3 module.networking.aws_route_table_association.tf_public_assoc.0: id = rtbassoc-0465f1772d010d6b5 route_table_id = rtb-079cac14ba01dbfc5 subnet_id = subnet-0e6566095894ef715 module.networking.aws_route_table_association.tf_public_assoc.1: id = rtbassoc-0c2b91ef65d48f1c9 route_table_id = rtb-079cac14ba01dbfc5 subnet_id = subnet-0cca01a76090c47be module.networking.aws_security_group.tf_public_sg: id = sg-0c7ed456b9ed183c9 arn = arn:aws:ec2:us-west-2:124011853020:security-group/sg-0c7ed456b9ed183c9 description = Used for access to the public instances egress.# = 1 egress.482069346.cidr_blocks.# = 1 egress.482069346.cidr_blocks.0 = 0.0.0.0/0 egress.482069346.description = egress.482069346.from_port = 0 egress.482069346.ipv6_cidr_blocks.# = 0 egress.482069346.prefix_list_ids.# = 0 egress.482069346.protocol = -1 egress.482069346.security_groups.# = 0 egress.482069346.self = false egress.482069346.to_port = 0 ingress.# = 2 ingress.2214680975.cidr_blocks.# = 1 ingress.2214680975.cidr_blocks.0 = 0.0.0.0/0 ingress.2214680975.description = ingress.2214680975.from_port = 80 ingress.2214680975.ipv6_cidr_blocks.# = 0 ingress.2214680975.prefix_list_ids.# = 0 ingress.2214680975.protocol = tcp ingress.2214680975.security_groups.# = 0 ingress.2214680975.self = false ingress.2214680975.to_port = 80 ingress.2541437006.cidr_blocks.# = 1 ingress.2541437006.cidr_blocks.0 = 0.0.0.0/0 ingress.2541437006.description = ingress.2541437006.from_port = 22 ingress.2541437006.ipv6_cidr_blocks.# = 0 ingress.2541437006.prefix_list_ids.# = 0 ingress.2541437006.protocol = tcp ingress.2541437006.security_groups.# = 0 ingress.2541437006.self = false ingress.2541437006.to_port = 22 name = tf_public_sg owner_id = 124011853020 revoke_rules_on_delete = false tags.% = 0 vpc_id = vpc-05498c93b1d9a8ab3 module.networking.aws_subnet.tf_public_subnet.0: id = subnet-0e6566095894ef715 arn = arn:aws:ec2:us-west-2:124011853020:subnet/subnet-0e6566095894ef715 assign_ipv6_address_on_creation = false availability_zone = us-west-2a cidr_block = 10.123.1.0/24 map_public_ip_on_launch = true tags.% = 1 tags.Name = tf_public_1 vpc_id = vpc-05498c93b1d9a8ab3 module.networking.aws_subnet.tf_public_subnet.1: id = subnet-0cca01a76090c47be arn = arn:aws:ec2:us-west-2:124011853020:subnet/subnet-0cca01a76090c47be assign_ipv6_address_on_creation = false availability_zone = us-west-2b cidr_block = 10.123.2.0/24 map_public_ip_on_launch = true tags.% = 1 tags.Name = tf_public_2 vpc_id = vpc-05498c93b1d9a8ab3 module.networking.aws_vpc.tf_vpc: id = vpc-05498c93b1d9a8ab3 arn = arn:aws:ec2:us-west-2:124011853020:vpc/vpc-05498c93b1d9a8ab3 assign_generated_ipv6_cidr_block = false cidr_block = 10.123.0.0/16 default_network_acl_id = acl-0166c532c42803834 default_route_table_id = rtb-0aea30b4837134bea default_security_group_id = sg-06867fd8132783af2 dhcp_options_id = dopt-cc5342a9 enable_classiclink = false enable_classiclink_dns_support = false enable_dns_hostnames = true enable_dns_support = true instance_tenancy = default main_route_table_id = rtb-0aea30b4837134bea tags.% = 1 tags.Name = tf_vpc module.networking.data.aws_availability_zones.available: id = 2018-10-06 14:58:22.628924121 +0000 UTC names.# = 3 names.0 = us-west-2a names.1 = us-west-2b names.2 = us-west-2c module.storage.aws_s3_bucket.tf_code: id = la-terrafrom-15307 acceleration_status = acl = private arn = arn:aws:s3:::la-terrafrom-15307 bucket = la-terrafrom-15307 bucket_domain_name = la-terrafrom-15307.s3.amazonaws.com bucket_regional_domain_name = la-terrafrom-15307.s3.us-west-2.amazonaws.com cors_rule.# = 0 force_destroy = true hosted_zone_id = Z3BJ6K6RIION7M lifecycle_rule.# = 0 logging.# = 0 region = us-west-2 replication_configuration.# = 0 request_payer = BucketOwner server_side_encryption_configuration.# = 0 tags.% = 1 tags.Name = tf_bucket versioning.# = 1 versioning.0.enabled = false versioning.0.mfa_delete = false website.# = 0 module.storage.random_id.tf_bucket_id: id = O8s b64 = O8s b64_std = O8s= b64_url = O8s byte_length = 2 dec = 15307 hex = 3bcb Outputs: Bucket Name = la-terrafrom-15307 Public Instance IDs = i-0c881f15e939d1bb6, i-00a1b4e02788b49e9 Public Instance IPs = 34.215.49.88, 34.214.144.245 Public Security Group = sg-0c7ed456b9ed183c9 Public Subnets = subnet-0e6566095894ef715, subnet-0cca01a76090c47be Subnet IPs = 10.123.1.0/24, 10.123.2.0/24
ec2-user:~/environment/AWS $ terraform destroy
module.storage.random_id.tf_bucket_id: Refreshing state... [id=ZtM] module.networking.data.aws_availability_zones.available: Refreshing state... [id=2020-10-10 01:49:21.78689905 +0000 UTC] module.compute.data.aws_ami.server_ami: Refreshing state... [id=ami-01fee56b22f308154] module.networking.aws_vpc.tf_vpc: Refreshing state... [id=vpc-091e518843d6ecb04] module.compute.aws_key_pair.tf_auth: Refreshing state... [id=tf_key] module.storage.aws_s3_bucket.tf_code: Refreshing state... [id=la-terrafrom-26323] module.networking.aws_default_route_table.tf_private_rt: Refreshing state... [id=rtb-07627bcc649fe3634] module.networking.aws_internet_gateway.tf_internet_gateway: Refreshing state... [id=igw-00b4b209a7da74eaf] module.networking.aws_subnet.tf_public_subnet[0]: Refreshing state... [id=subnet-060302a46a656405b] module.networking.aws_security_group.tf_public_sg: Refreshing state... [id=sg-094bcb58948a6a96d] module.networking.aws_subnet.tf_public_subnet[1]: Refreshing state... [id=subnet-0fbcea1253364514c] module.networking.aws_route_table.tf_public_rt: Refreshing state... [id=rtb-0992d0e19d5dcbbe6] module.compute.data.template_file.user-init[1]: Refreshing state... [id=757bacc9f6377916bf38eff5b6acdb9ae2756c56859b9c203d8de26eff4865d4] module.compute.data.template_file.user-init[0]: Refreshing state... [id=57df141e108f0b6cf1703691b1a5a736ca3a01b0eb84e07196d778d405c36d19] module.networking.aws_route_table_association.tf_public_assoc[0]: Refreshing state... [id=rtbassoc-0ea0e00e4ef1ff3df] module.networking.aws_route_table_association.tf_public_assoc[1]: Refreshing state... [id=rtbassoc-0d79338bd4c127b42] module.compute.aws_instance.tf_server[1]: Refreshing state... [id=i-0186d81c4a1141e86] module.compute.aws_instance.tf_server[0]: Refreshing state... [id=i-02f7f327d22eb6dc6] An execution plan has been generated and is shown below. Resource actions are indicated with the following symbols: - destroy Terraform will perform the following actions: # module.compute.aws_instance.tf_server[0] will be destroyed - resource "aws_instance" "tf_server" { - ami = "ami-01fee56b22f308154" -> null - arn = "arn:aws:ec2:us-west-2:124011853020:instance/i-02f7f327d22eb6dc6" -> null - associate_public_ip_address = true -> null - availability_zone = "us-west-2a" -> null - cpu_core_count = 1 -> null - cpu_threads_per_core = 1 -> null - disable_api_termination = false -> null - ebs_optimized = false -> null - get_password_data = false -> null - hibernation = false -> null - id = "i-02f7f327d22eb6dc6" -> null - instance_state = "running" -> null - instance_type = "t2.micro" -> null - ipv6_address_count = 0 -> null - ipv6_addresses = [] -> null - key_name = "tf_key" -> null - monitoring = false -> null - primary_network_interface_id = "eni-05c1941948113009b" -> null - private_dns = "ip-10-123-1-150.us-west-2.compute.internal" -> null - private_ip = "10.123.1.150" -> null - public_dns = "ec2-54-214-69-170.us-west-2.compute.amazonaws.com" -> null - public_ip = "54.214.69.170" -> null - secondary_private_ips = [] -> null - security_groups = [] -> null - source_dest_check = true -> null - subnet_id = "subnet-060302a46a656405b" -> null - tags = { - "Name" = "tf_server-1" } -> null - tenancy = "default" -> null - user_data = "544105fc76f56380fa17cd0686e033cee5001d87" -> null - volume_tags = {} -> null - vpc_security_group_ids = [ - "sg-094bcb58948a6a96d", ] -> null - credit_specification { - cpu_credits = "standard" -> null } - metadata_options { - http_endpoint = "enabled" -> null - http_put_response_hop_limit = 1 -> null - http_tokens = "optional" -> null } - root_block_device { - delete_on_termination = true -> null - device_name = "/dev/xvda" -> null - encrypted = false -> null - iops = 100 -> null - volume_id = "vol-0ed8336a4bd536539" -> null - volume_size = 8 -> null - volume_type = "gp2" -> null } } # module.compute.aws_instance.tf_server[1] will be destroyed - resource "aws_instance" "tf_server" { - ami = "ami-01fee56b22f308154" -> null - arn = "arn:aws:ec2:us-west-2:124011853020:instance/i-0186d81c4a1141e86" -> null - associate_public_ip_address = true -> null - availability_zone = "us-west-2b" -> null - cpu_core_count = 1 -> null - cpu_threads_per_core = 1 -> null - disable_api_termination = false -> null - ebs_optimized = false -> null - get_password_data = false -> null - hibernation = false -> null - id = "i-0186d81c4a1141e86" -> null - instance_state = "running" -> null - instance_type = "t2.micro" -> null - ipv6_address_count = 0 -> null - ipv6_addresses = [] -> null - key_name = "tf_key" -> null - monitoring = false -> null - primary_network_interface_id = "eni-05cd8904109496549" -> null - private_dns = "ip-10-123-2-95.us-west-2.compute.internal" -> null - private_ip = "10.123.2.95" -> null - public_dns = "ec2-35-166-52-155.us-west-2.compute.amazonaws.com" -> null - public_ip = "35.166.52.155" -> null - secondary_private_ips = [] -> null - security_groups = [] -> null - source_dest_check = true -> null - subnet_id = "subnet-0fbcea1253364514c" -> null - tags = { - "Name" = "tf_server-2" } -> null - tenancy = "default" -> null - user_data = "ea5b38a77b74322af7f46802b7e74b3277c7eb0d" -> null - volume_tags = {} -> null - vpc_security_group_ids = [ - "sg-094bcb58948a6a96d", ] -> null - credit_specification { - cpu_credits = "standard" -> null } - metadata_options { - http_endpoint = "enabled" -> null - http_put_response_hop_limit = 1 -> null - http_tokens = "optional" -> null } - root_block_device { - delete_on_termination = true -> null - device_name = "/dev/xvda" -> null - encrypted = false -> null - iops = 100 -> null - volume_id = "vol-03211f99c46070a7c" -> null - volume_size = 8 -> null - volume_type = "gp2" -> null } } # module.compute.aws_key_pair.tf_auth will be destroyed - resource "aws_key_pair" "tf_auth" { - arn = "arn:aws:ec2:us-west-2:124011853020:key-pair/tf_key" -> null - fingerprint = "9d:54:ea:2a:70:a8:52:d7:4c:56:16:0f:8b:83:2e:3b" -> null - id = "tf_key" -> null - key_name = "tf_key" -> null - key_pair_id = "key-0fe18333b70817847" -> null - public_key = "ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQCzIeDhLeOVMfyYVV4ePCm/X4uRRmqkqq84TU2VsBDHWtMuFwBbez2ZmAm+WQ5zOyaZC/soSK17R8TsociZ+9wJBWT62aS3H8IHE2UxoakjlucF1QLM81oZaO5R4DCeKVJb0l/XfZ/fQkhYFLNM5622MbHP8MTTwfrbwE1+hjRFJYb0K364NCD0BLdgn+V7kfyEcSRRds8gh8zdiejJxFHRTaq9LRx+AQwFDkQYEYzk6ZGxIasKonCD18OtwAePdFgA1Mlho6Ajh9VyrgYWrEHKmfvDa/Rz7T/cCy5tkzdu5B04HWI7yBEthZeKm9QA8keOj1xU+yMWSNwUhiXGTg85 ec2-user@ip-10-0-0-44.us-west-2.compute.internal" -> null - tags = {} -> null } # module.networking.aws_default_route_table.tf_private_rt will be destroyed - resource "aws_default_route_table" "tf_private_rt" { - default_route_table_id = "rtb-07627bcc649fe3634" -> null - id = "rtb-07627bcc649fe3634" -> null - owner_id = "124011853020" -> null - propagating_vgws = [] -> null - route = [] -> null - tags = { - "Name" = "tf_private" } -> null - vpc_id = "vpc-091e518843d6ecb04" -> null } # module.networking.aws_internet_gateway.tf_internet_gateway will be destroyed - resource "aws_internet_gateway" "tf_internet_gateway" { - arn = "arn:aws:ec2:us-west-2:124011853020:internet-gateway/igw-00b4b209a7da74eaf" -> null - id = "igw-00b4b209a7da74eaf" -> null - owner_id = "124011853020" -> null - tags = { - "Name" = "tf_igw" } -> null - vpc_id = "vpc-091e518843d6ecb04" -> null } # module.networking.aws_route_table.tf_public_rt will be destroyed - resource "aws_route_table" "tf_public_rt" { - id = "rtb-0992d0e19d5dcbbe6" -> null - owner_id = "124011853020" -> null - propagating_vgws = [] -> null - route = [ - { - cidr_block = "0.0.0.0/0" - egress_only_gateway_id = "" - gateway_id = "igw-00b4b209a7da74eaf" - instance_id = "" - ipv6_cidr_block = "" - local_gateway_id = "" - nat_gateway_id = "" - network_interface_id = "" - transit_gateway_id = "" - vpc_peering_connection_id = "" }, ] -> null - tags = { - "Name" = "tf_public" } -> null - vpc_id = "vpc-091e518843d6ecb04" -> null } # module.networking.aws_route_table_association.tf_public_assoc[0] will be destroyed - resource "aws_route_table_association" "tf_public_assoc" { - id = "rtbassoc-0ea0e00e4ef1ff3df" -> null - route_table_id = "rtb-0992d0e19d5dcbbe6" -> null - subnet_id = "subnet-060302a46a656405b" -> null } # module.networking.aws_route_table_association.tf_public_assoc[1] will be destroyed - resource "aws_route_table_association" "tf_public_assoc" { - id = "rtbassoc-0d79338bd4c127b42" -> null - route_table_id = "rtb-0992d0e19d5dcbbe6" -> null - subnet_id = "subnet-0fbcea1253364514c" -> null } # module.networking.aws_security_group.tf_public_sg will be destroyed - resource "aws_security_group" "tf_public_sg" { - arn = "arn:aws:ec2:us-west-2:124011853020:security-group/sg-094bcb58948a6a96d" -> null - description = "Used for access to the public instances" -> null - egress = [ - { - cidr_blocks = [ - "0.0.0.0/0", ] - description = "" - from_port = 0 - ipv6_cidr_blocks = [] - prefix_list_ids = [] - protocol = "-1" - security_groups = [] - self = false - to_port = 0 }, ] -> null - id = "sg-094bcb58948a6a96d" -> null - ingress = [ - { - cidr_blocks = [ - "0.0.0.0/0", ] - description = "" - from_port = 22 - ipv6_cidr_blocks = [] - prefix_list_ids = [] - protocol = "tcp" - security_groups = [] - self = false - to_port = 22 }, - { - cidr_blocks = [ - "0.0.0.0/0", ] - description = "" - from_port = 80 - ipv6_cidr_blocks = [] - prefix_list_ids = [] - protocol = "tcp" - security_groups = [] - self = false - to_port = 80 }, ] -> null - name = "tf_public_sg" -> null - owner_id = "124011853020" -> null - revoke_rules_on_delete = false -> null - tags = {} -> null - vpc_id = "vpc-091e518843d6ecb04" -> null } # module.networking.aws_subnet.tf_public_subnet[0] will be destroyed - resource "aws_subnet" "tf_public_subnet" { - arn = "arn:aws:ec2:us-west-2:124011853020:subnet/subnet-060302a46a656405b" -> null - assign_ipv6_address_on_creation = false -> null - availability_zone = "us-west-2a" -> null - availability_zone_id = "usw2-az1" -> null - cidr_block = "10.123.1.0/24" -> null - id = "subnet-060302a46a656405b" -> null - map_public_ip_on_launch = true -> null - owner_id = "124011853020" -> null - tags = { - "Name" = "tf_public_1" } -> null - vpc_id = "vpc-091e518843d6ecb04" -> null } # module.networking.aws_subnet.tf_public_subnet[1] will be destroyed - resource "aws_subnet" "tf_public_subnet" { - arn = "arn:aws:ec2:us-west-2:124011853020:subnet/subnet-0fbcea1253364514c" -> null - assign_ipv6_address_on_creation = false -> null - availability_zone = "us-west-2b" -> null - availability_zone_id = "usw2-az2" -> null - cidr_block = "10.123.2.0/24" -> null - id = "subnet-0fbcea1253364514c" -> null - map_public_ip_on_launch = true -> null - owner_id = "124011853020" -> null - tags = { - "Name" = "tf_public_2" } -> null - vpc_id = "vpc-091e518843d6ecb04" -> null } # module.networking.aws_vpc.tf_vpc will be destroyed - resource "aws_vpc" "tf_vpc" { - arn = "arn:aws:ec2:us-west-2:124011853020:vpc/vpc-091e518843d6ecb04" -> null - assign_generated_ipv6_cidr_block = false -> null - cidr_block = "10.123.0.0/16" -> null - default_network_acl_id = "acl-0cdb8cb004bdfa1ec" -> null - default_route_table_id = "rtb-07627bcc649fe3634" -> null - default_security_group_id = "sg-022aeddefab97e010" -> null - dhcp_options_id = "dopt-cc5342a9" -> null - enable_classiclink = false -> null - enable_classiclink_dns_support = false -> null - enable_dns_hostnames = true -> null - enable_dns_support = true -> null - id = "vpc-091e518843d6ecb04" -> null - instance_tenancy = "default" -> null - main_route_table_id = "rtb-07627bcc649fe3634" -> null - owner_id = "124011853020" -> null - tags = { - "Name" = "tf_vpc" } -> null } # module.storage.aws_s3_bucket.tf_code will be destroyed - resource "aws_s3_bucket" "tf_code" { - acl = "private" -> null - arn = "arn:aws:s3:::la-terrafrom-26323" -> null - bucket = "la-terrafrom-26323" -> null - bucket_domain_name = "la-terrafrom-26323.s3.amazonaws.com" -> null - bucket_regional_domain_name = "la-terrafrom-26323.s3.us-west-2.amazonaws.com" -> null - force_destroy = true -> null - hosted_zone_id = "Z3BJ6K6RIION7M" -> null - id = "la-terrafrom-26323" -> null - region = "us-west-2" -> null - request_payer = "BucketOwner" -> null - tags = { - "Name" = "tf_bucket" } -> null - versioning { - enabled = false -> null - mfa_delete = false -> null } } # module.storage.random_id.tf_bucket_id will be destroyed - resource "random_id" "tf_bucket_id" { - b64_std = "ZtM=" -> null - b64_url = "ZtM" -> null - byte_length = 2 -> null - dec = "26323" -> null - hex = "66d3" -> null - id = "ZtM" -> null } Plan: 0 to add, 0 to change, 14 to destroy. Changes to Outputs: - Bucket_Name = "la-terrafrom-26323" -> null - Public_Instance_IDs = "i-02f7f327d22eb6dc6, i-0186d81c4a1141e86" -> null - Public_Instance_IPs = "54.214.69.170, 35.166.52.155" -> null - Public_Security_Group = "sg-094bcb58948a6a96d" -> null - Public_Subnets = "subnet-060302a46a656405b, subnet-0fbcea1253364514c" -> null - Subnet_IPs = "10.123.1.0/24, 10.123.2.0/24" -> null Do you really want to destroy all resources? Terraform will destroy all your managed infrastructure, as shown above. There is no undo. Only 'yes' will be accepted to confirm. Enter a value: yes module.networking.aws_route_table_association.tf_public_assoc[0]: Destroying... [id=rtbassoc-0ea0e00e4ef1ff3df] module.networking.aws_route_table_association.tf_public_assoc[1]: Destroying... [id=rtbassoc-0d79338bd4c127b42] module.compute.aws_instance.tf_server[0]: Destroying... [id=i-02f7f327d22eb6dc6] module.storage.aws_s3_bucket.tf_code: Destroying... [id=la-terrafrom-26323] module.networking.aws_default_route_table.tf_private_rt: Destroying... [id=rtb-07627bcc649fe3634] module.compute.aws_instance.tf_server[1]: Destroying... [id=i-0186d81c4a1141e86] module.networking.aws_default_route_table.tf_private_rt: Destruction complete after 0s module.networking.aws_route_table_association.tf_public_assoc[0]: Destruction complete after 1s module.networking.aws_route_table_association.tf_public_assoc[1]: Destruction complete after 1s module.networking.aws_route_table.tf_public_rt: Destroying... [id=rtb-0992d0e19d5dcbbe6] module.storage.aws_s3_bucket.tf_code: Destruction complete after 1s module.storage.random_id.tf_bucket_id: Destroying... [id=ZtM] module.storage.random_id.tf_bucket_id: Destruction complete after 0s module.networking.aws_route_table.tf_public_rt: Destruction complete after 0s module.networking.aws_internet_gateway.tf_internet_gateway: Destroying... [id=igw-00b4b209a7da74eaf] module.compute.aws_instance.tf_server[0]: Still destroying... [id=i-02f7f327d22eb6dc6, 10s elapsed] module.compute.aws_instance.tf_server[1]: Still destroying... [id=i-0186d81c4a1141e86, 10s elapsed] module.networking.aws_internet_gateway.tf_internet_gateway: Still destroying... [id=igw-00b4b209a7da74eaf, 10s elapsed] module.compute.aws_instance.tf_server[0]: Still destroying... [id=i-02f7f327d22eb6dc6, 20s elapsed] module.compute.aws_instance.tf_server[1]: Still destroying... [id=i-0186d81c4a1141e86, 20s elapsed] module.networking.aws_internet_gateway.tf_internet_gateway: Still destroying... [id=igw-00b4b209a7da74eaf, 20s elapsed] module.compute.aws_instance.tf_server[0]: Still destroying... [id=i-02f7f327d22eb6dc6, 30s elapsed] module.compute.aws_instance.tf_server[1]: Still destroying... [id=i-0186d81c4a1141e86, 30s elapsed] module.networking.aws_internet_gateway.tf_internet_gateway: Still destroying... [id=igw-00b4b209a7da74eaf, 30s elapsed] module.networking.aws_internet_gateway.tf_internet_gateway: Destruction complete after 34s module.compute.aws_instance.tf_server[1]: Destruction complete after 40s module.compute.aws_instance.tf_server[0]: Destruction complete after 40s module.networking.aws_subnet.tf_public_subnet[1]: Destroying... [id=subnet-0fbcea1253364514c] module.networking.aws_security_group.tf_public_sg: Destroying... [id=sg-094bcb58948a6a96d] module.compute.aws_key_pair.tf_auth: Destroying... [id=tf_key] module.networking.aws_subnet.tf_public_subnet[0]: Destroying... [id=subnet-060302a46a656405b] module.compute.aws_key_pair.tf_auth: Destruction complete after 0s module.networking.aws_security_group.tf_public_sg: Destruction complete after 0s module.networking.aws_subnet.tf_public_subnet[1]: Destruction complete after 1s module.networking.aws_subnet.tf_public_subnet[0]: Destruction complete after 1s module.networking.aws_vpc.tf_vpc: Destroying... [id=vpc-091e518843d6ecb04] module.networking.aws_vpc.tf_vpc: Destruction complete after 0s Destroy complete! Resources: 14 destroyed.
References
Command: fmt